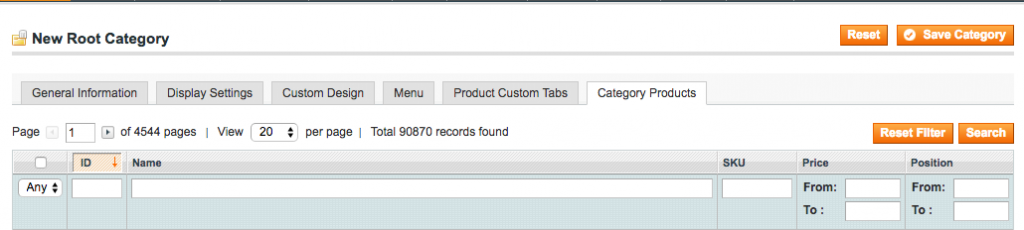
Recently I discovered all the product positions after being assigned to a category were defaulted to 1. I needed to change this number to anything other than 1.
I found that Magento currently defaults this number to 1 in their resource model under the function _saveCategories. The entire class would be Mage_Catalog_Model_Resource_Product.
$data[] = array(
'category_id' => (int)$categoryId,
'product_id' => (int)$object->getId(),
'position' => 1
);
In order to solve this problem, I decided to do a quick rewrite of this resource model. You should rewrite this class in your modules config file like so.
<models>
<module_name>
<class>Module_Name_Model</class>
</module_name>
<catalog_resource>
<rewrite>
<product>Module_Name_Model_Catalog_Resource_Product</product>
</rewrite>
</catalog_resource>
</models>
Then create the file Module_Name_Model_Catalog_Resource_Product and extend Mage_Catalog_Model_Resource_Product
class Module_Name_Model_Catalog_Resource_Product extends Mage_Catalog_Model_Resource_Product {
/**
* Save product category relations
*
* @param Varien_Object $object
* @return Mage_Catalog_Model_Resource_Product
*/
protected function _saveCategories(Varien_Object $object)
{
/**
* If category ids data is not declared we haven't do manipulations
*/
if (!$object->hasCategoryIds()) {
return $this;
}
$categoryIds = $object->getCategoryIds();
$oldCategoryIds = $this->getCategoryIds($object);
$object->setIsChangedCategories(false);
$insert = array_diff($categoryIds, $oldCategoryIds);
$delete = array_diff($oldCategoryIds, $categoryIds);
$write = $this->_getWriteAdapter();
if (!empty($insert)) {
$data = array();
foreach ($insert as $categoryId) {
if (empty($categoryId)) {
continue;
}
$data[] = array(
'category_id' => (int)$categoryId,
'product_id' => (int)$object->getId(),
'position' => 9999
);
}
if ($data) {
$write->insertMultiple($this->_productCategoryTable, $data);
}
}
if (!empty($delete)) {
foreach ($delete as $categoryId) {
$where = array(
'product_id = ?' => (int)$object->getId(),
'category_id = ?' => (int)$categoryId,
);
$write->delete($this->_productCategoryTable, $where);
}
}
if (!empty($insert) || !empty($delete)) {
$object->setAffectedCategoryIds(array_merge($insert, $delete));
$object->setIsChangedCategories(true);
}
return $this;
}
}
Show us some ❤️
If you found this article useful and informative, please share it with others who may benefit from it. Also, feel free to leave a comment below with your thoughts or any questions you may have.